NodeJS
like this picture, you can routing page with url easily.
app.js
main.html
Internet browser
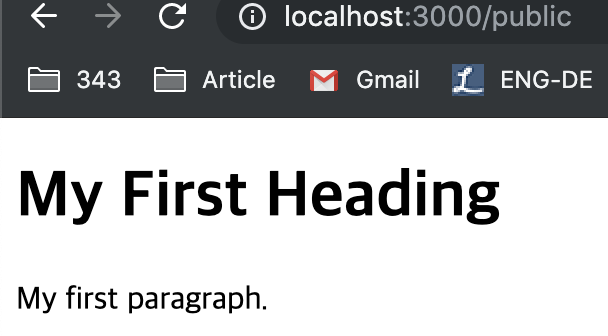
Also, if you use “app.use” function, server set public folder as a static.
var express = require('express')
var app = express()
app.listen(3000, function() {
console.log("start!! express server on port 3000");
});
//function app.use
app.use(express.static('public'))
//url routing
app.get('/', function(req,res){
res.sendFile(__dirname + '/public/main.html')
});
app.get('/main', function(req,res){
res.sendFile(__dirname + '/public/main.html')
});
Continue reading
NodeJS
First of all, to parse data, we need to install body-parser
npm install body-parser --save
form.html
<!DOCTYPE html>
<html>
<head>
<meta charset="uft-8">
<title>email form</title>
<link rel="stylesheet" href="css/style.css">
<link rel="author" href="humans.txt">
</head>
<body>
<form action="/email_post" method="post">
email : <input type="text" name="email"><br/>
submit <input type="submit">
</form>
<script src="js/main.js"></script>
</body>
</html>
app.js
var express = require('express')
var app = express()
// declare bodyParser like as above code.
var bodyParser = require('body-parser')
app.listen(3000, function() {
console.log("start!! express server on port 3000");
});
app.use(express.static('public'))
// code could be json style
app.use(bodyParser.json())
// code could be encoded
app.use(bodyParser.urlencoded({extended:true}))
//url routing
app.get('/', function(req,res){
res.sendFile(__dirname + '/public/main.html')
});
app.get('/main', function(req,res){
res.sendFile(__dirname + '/public/main.html')
});
// if any page call the /email_post
// server will print req.body.email in console
// in case of form.html, the information should be content in input text for e-mail form
app.post ('/email_post', function(req,res){
//get : req.parm('email')
console.log(req.body.email)
res.send("post response");
});
Continue reading
jQuery
You can have so many plugin for JQuery.
jstree help you to make a tree structure quickly.
https://www.jstree.com/
jstree test using ajax
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Insert title here</title>
<script type="text/ecmascript" src="/jquery/jquery-1.12.4.min.js"></script>
<script type="text/ecmascript" src="/jquery/jstree/jstree.min.js"></script>
<link rel="stylesheet" type="text/css" href="/jquery/jstree/themes/default/style.min.css" />
<script type="text/javascript">
$(function () {
$('#jstree').jstree({
'core' : {
'data' : [
'Simple root node',
{
'text' : 'Root node 2',
'state' : {
'opened' : true,
'selected' : true
},
'children' : [
{ 'text' : 'Child 1' },
{ 'text' : 'Child 2'}]
}
]
} });
})
</script>
</head>
<body>
<div id="jstree">
</div>
</body>
</html>
Continue reading